Overview
import CustomListView from "@components/custom-list-view/CustomListView"
;
Description
this component allows to show an array in a list view
it supports filtering and pagination out of the box
Usage example
Code
import CustomListView from "@components/custom-list-view/CustomListView";
function Demo() {
let data = [
{
id: "1",
title: "element 1",
src: "https://images.pexels.com/photos/39853/woman-girl-freedom-happy-39853.jpeg",
},
{
id: "2",
title: "element 2",
src: "https://images.pexels.com/photos/2486186/pexels-photo-2486186.jpeg",
},
{
id: "3",
title: "element 3",
src: "https://images.pexels.com/photos/318420/pexels-photo-318420.jpeg",
},
{
id: "4",
title: "element 4",
src: "https://images.pexels.com/photos/1366630/pexels-photo-1366630.jpeg",
},
{
id: "5",
title: "element 5",
src: "https://images.pexels.com/photos/13950012/pexels-photo-13950012.jpeg",
},
];
return (
<CustomListView
data={data}
renderItem={(element) => (
<div className="list-item">
<span>
<img src={element.src} />
</span>
<span className="list-item-title"> {element.title}</span>
</div>
)}
/>
);
}
Result
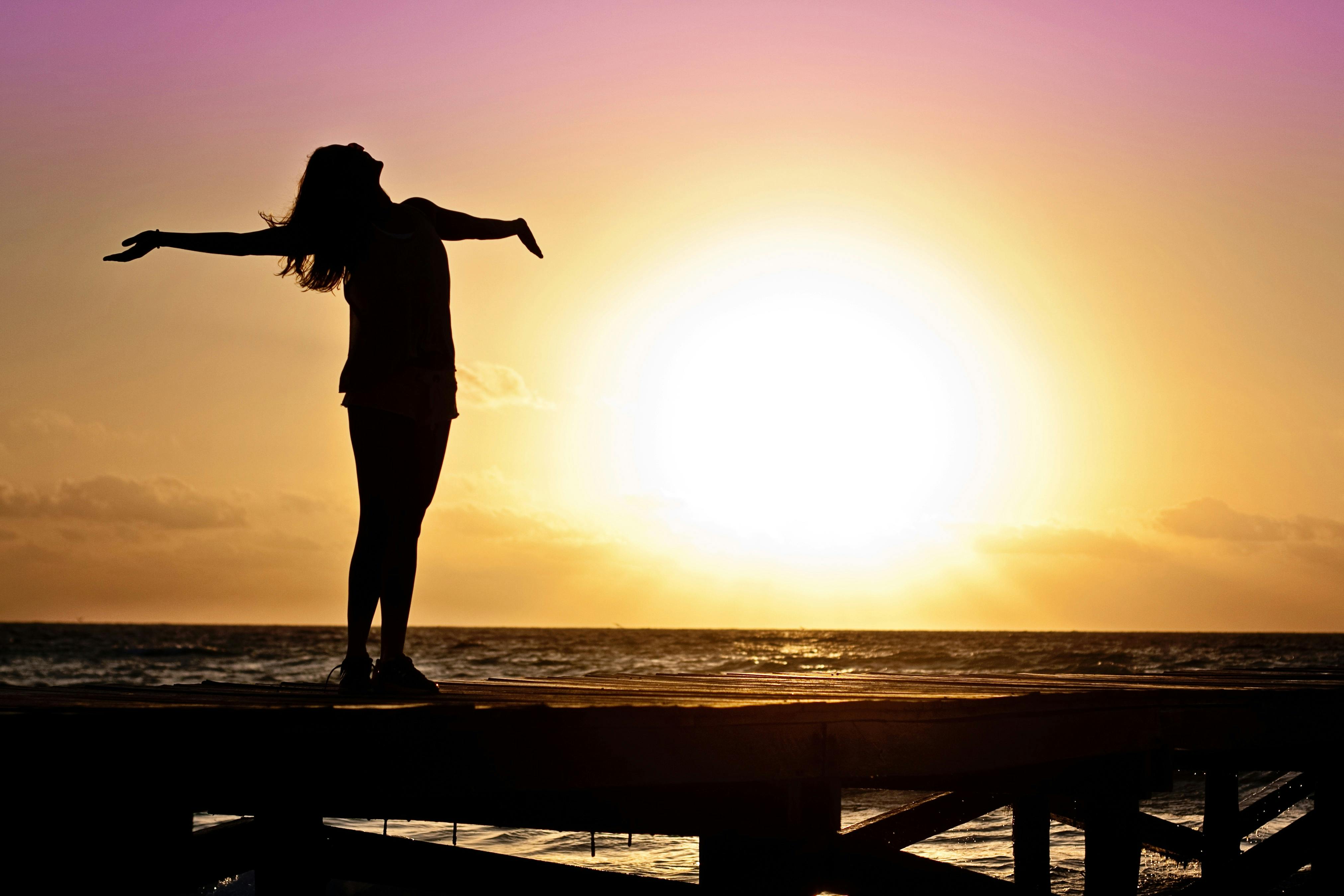

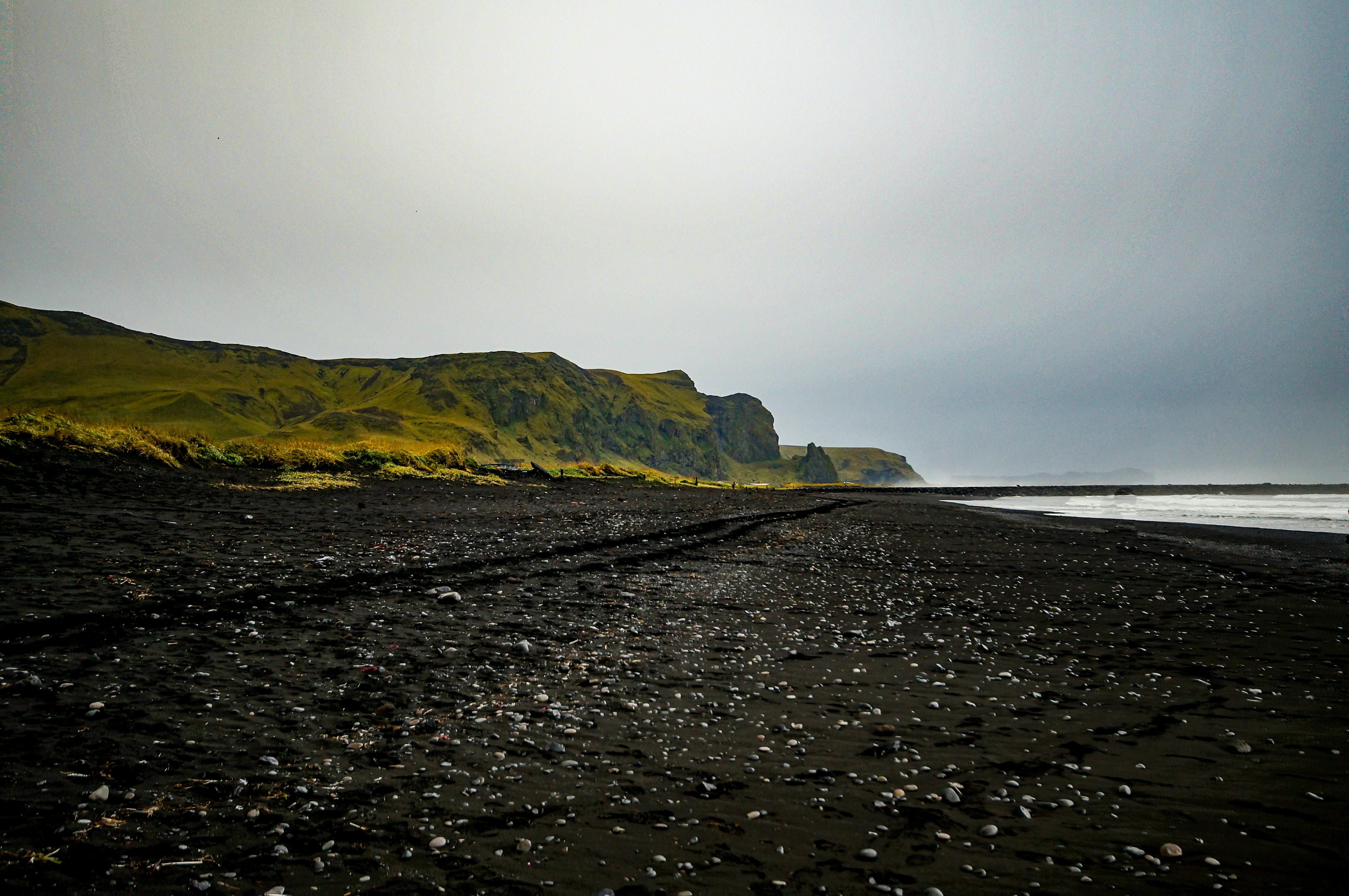
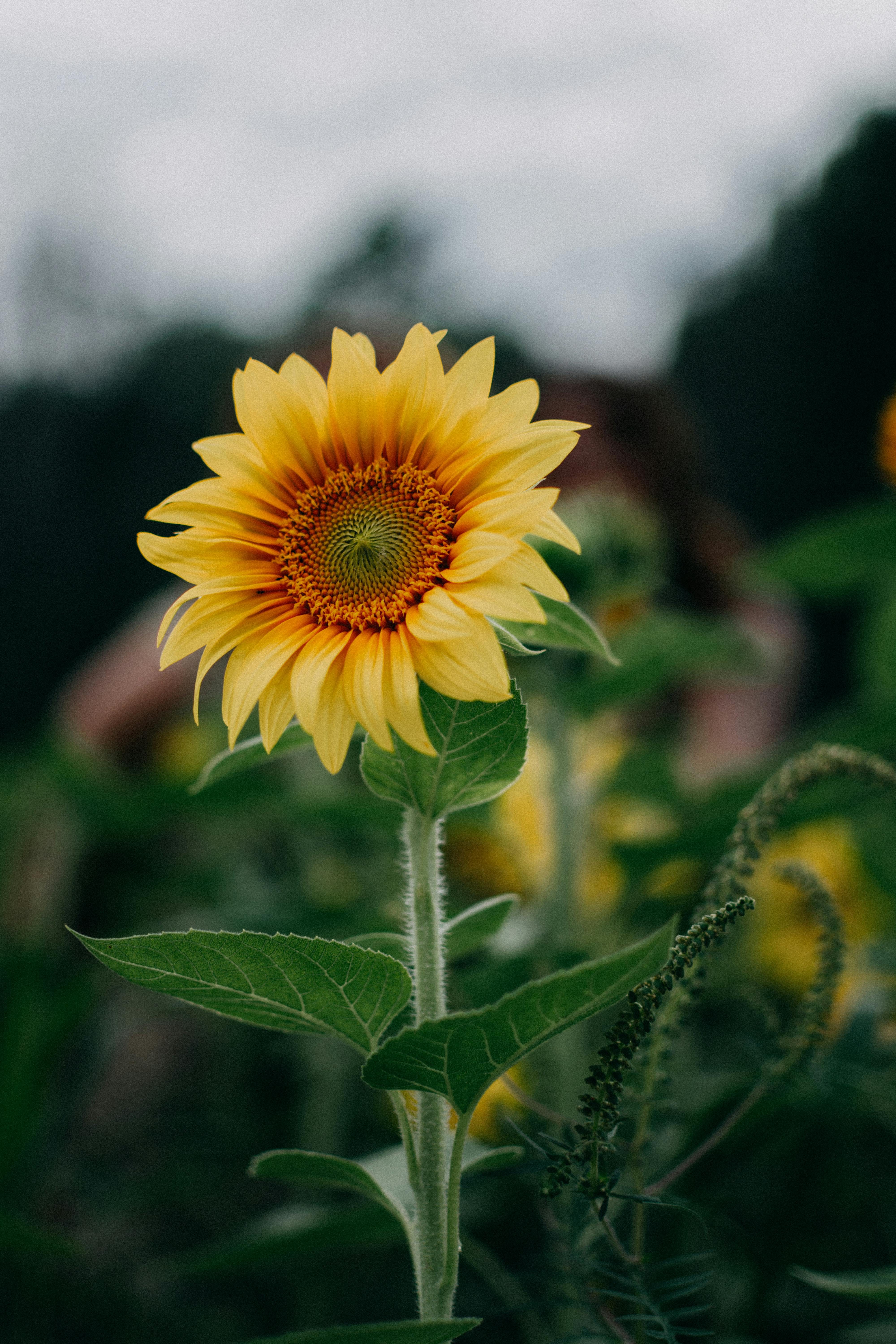

Props
Name | Type | Description |
---|---|---|
data | array | the list of elements to be rendered |
initialDataState | DataState | the state to be applied on data |
renderItem | Component | the Component in which each item will be rendered |